This post is for both the junior developer just getting started and the seasoned pro with years of experience. In my time working with devs at all levels, one thing remains true: we’re all still learning. So let’s take a moment to go back to basics. Sometimes, the simplest approach is the best—especially when “best” means saving time and effort. That mindset is something I’ve consistently seen in professionals and business owners deeply immersed in tech and development.
In fact, I’ve had both a developer with over a decade of experience and someone brand new to the field ask me the same thing:
“What’s the quickest way to start testing an idea or building something out?”
Here’s my take.
Web development is a massive, ever-evolving space. Just look at how Web 3.0 is reshaping infrastructure. With that growth comes an overwhelming number of tools. Many are powerful, and I’ll mention a few that stand out—but often, all those extra features can become… extra. Sometimes you don’t need the full suite; sometimes you just want to start building.
Before we simplify it further, a quick mention of some helpful tools
Frontend sandboxes like CodePen, JSFiddle, JSBin, CSSDeck, and Dabblet are perfect for experimenting with HTML, CSS, and JavaScript in real time. They offer live previews, easy sharing, and help you quickly prototype ideas or test visual changes.
For more complex or framework-heavy work, there are full-stack environments like:
- CodeSandbox – Great for working with React, Vue, and npm packages.
- StackBlitz – A browser-based IDE that shines with Angular and TypeScript.
- Replit – Supports 50+ languages, real-time collaboration, and backend logic.
These platforms are incredibly useful—but truthfully, for a lot of use cases, they’re more than you need. Sometimes you just want to lay out your page, test an idea, and not get bogged down with tools and settings.
That’s why I always advocate for starting as simple as possible. Simplicity focuses resource on the idea itself—not the setup.
Back to Basics: One File, Real-Time Results
Let’s talk about spinning up a webpage with just one file—no installs, no builds, just code and go. This method lets you prototype freely while still being flexible enough to support powerful libraries like React, Vue.js, or Bootstrap if and when you need them.
For experienced devs, I’d still recommend using a code editor like Visual Studio Code paired with a tool like Live Server for a smoother development experience—but that’s a bonus, not a requirement. You don’t need anything fancy to get started.
The steps I’ll show are on Windows, using Notepad (a Windows pre-installed text editor) but the concept applies universally across systems.
Let’s dive in.
Make an HTML file called KeepItSimpleSophocles.html
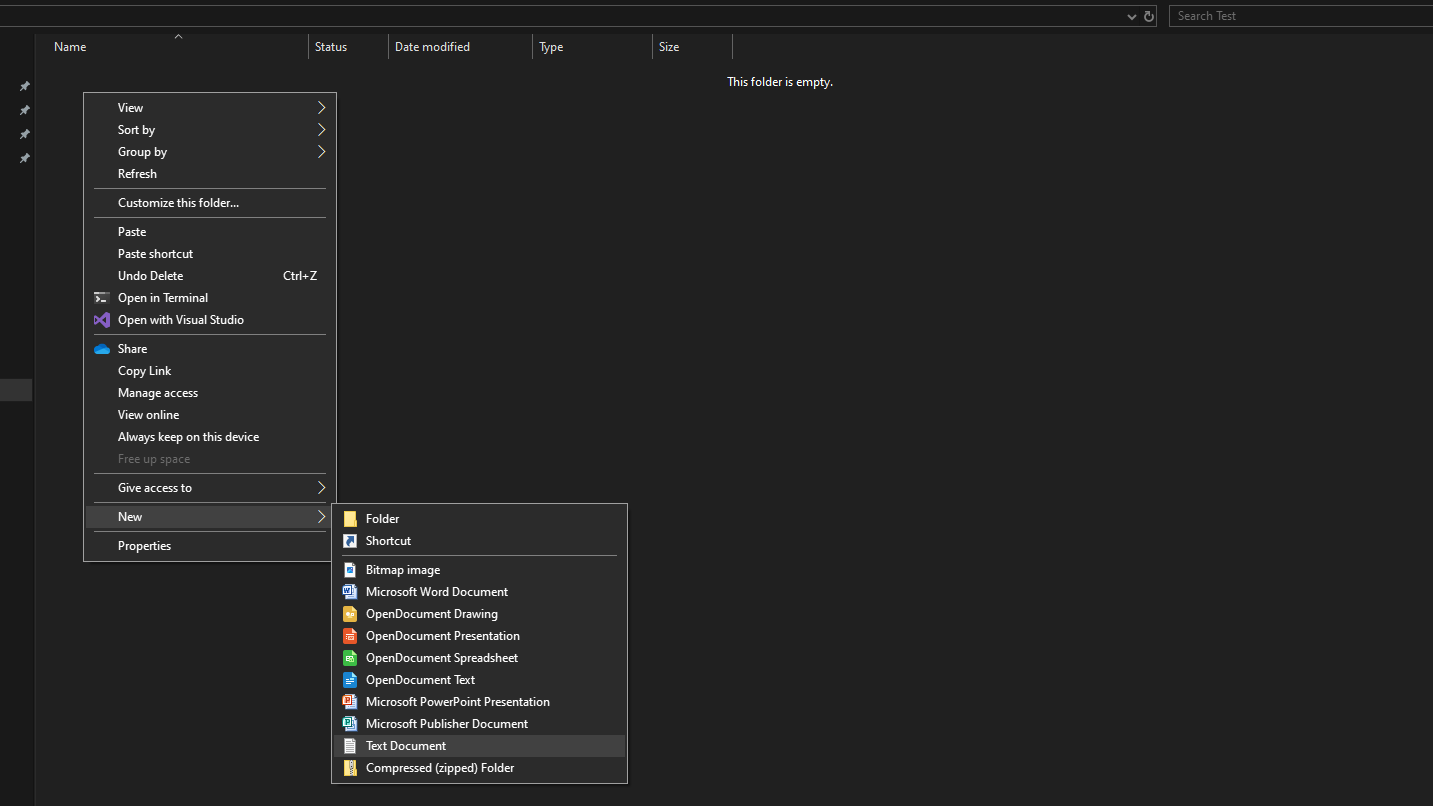
Step 1: Right click in any directory or on desktop. Hover over New. Click Text Document. Give it a name like “KeepItSimpleSophocles” and change the extension to .html while at it.
Open the file with a text editor such as Notepad (VS Code or Notepad++ are great alternatives)
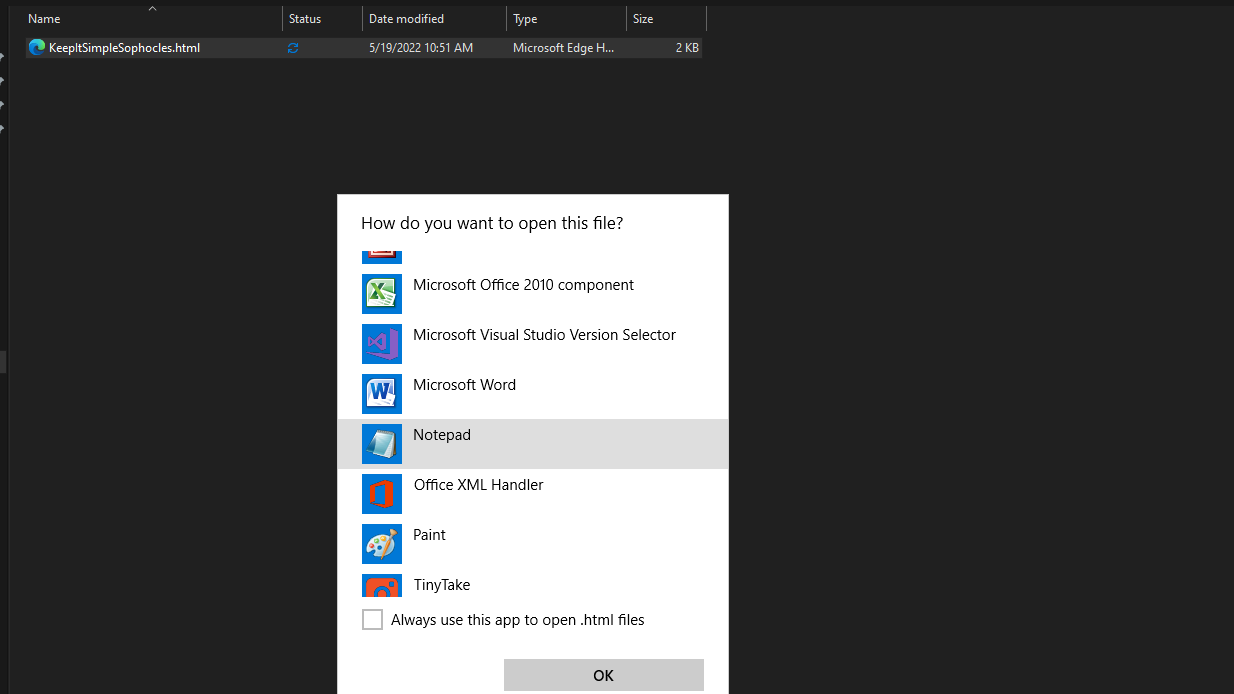
Step 2: If you changed the file type to .html as the previous step indicated then you should right click on the file and click Open With -> Select Notepad if it is present otherwise click Open With Other App and browse for and select the Notepad application.
Add some html content to the file
<!DOCTYPE html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title></title>
<meta name="description" content="">
<link rel="shortcut icon" href="favicon.ico">
<style>
</style>
</head>
<body>
<h2>"The greatest griefs are those we cause ourselves."</h2>
<p>-Keepin' It Simple Sophocles (KISS)</p>
<script>
</script>
</body>
</html>
Step 3: Copy the html template above and paste into your file opened with Notepad. Save the file. Double click on the file and your computer’s default browser should open the file up. If it doesn’t then simply browse to open the the file with the browser: Right Click file -> Open With -> Select Chrome (or any browser) if it is present otherwise click Open With Other App and browse for and select the Chrome application. You should see a webpage display similar to the following:
“The greatest griefs are those we cause ourselves.”
-Keepin’ It Simple Sophocles (KISS)